8. For student and course models created in Lab experiment for Module2, register admin interfaces, perform migrations and illustrate data entry through admin forms.
Step-01: Create new folder:-
⦿ In the same project folder whatever we made earlier create again one new app name called as ‘registration’ using below command.
cd project
python manage.py startapp registration
Step-02: Add the ‘registration’ to the installed_apps list:-
⦿ locate the settings.py file (usually located in the project directory) and open it.
⦿After then add your app name in install_apps list as per below image.
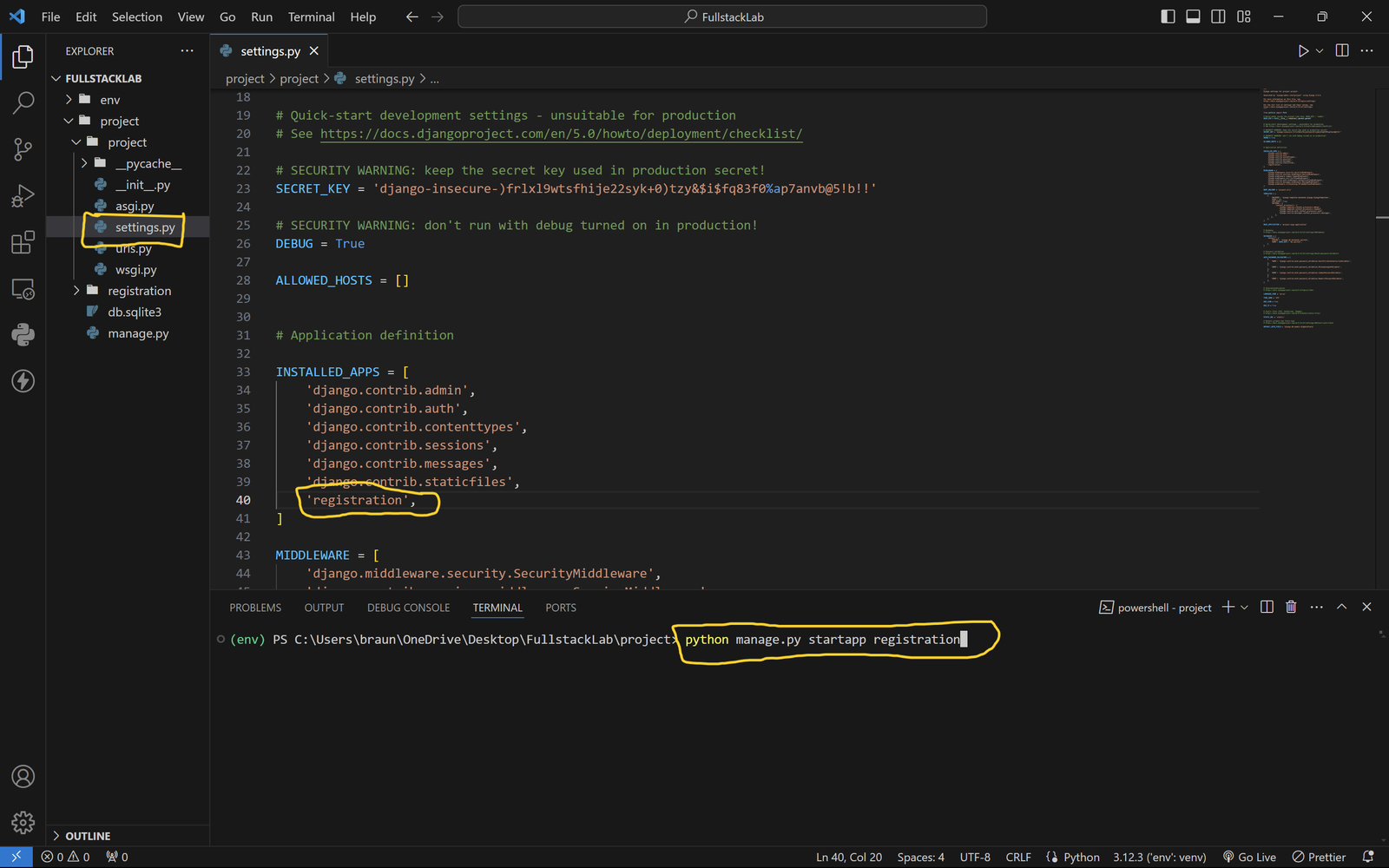
Step-03: Inside models.py file create a model:-
from django.db import models
class Course(models.Model):
name = models.CharField(max_length=100)
description = models.TextField()
def __str__(self):
return self.name
@property
def student_count(self):
return self.students.count()
class Student(models.Model):
first_name = models.CharField(max_length=50)
last_name = models.CharField(max_length=50)
email = models.EmailField(unique=True, null=True)
phone_number = models.CharField(max_length=15, null=True)
courses = models.ManyToManyField(Course, related_name='students', through='Enrollment')
def __str__(self):
return f"{self.first_name} {self.last_name}"
class Enrollment(models.Model):
student = models.ForeignKey(Student, on_delete=models.CASCADE)
course = models.ForeignKey(Course, on_delete=models.CASCADE)
enrolled_on = models.DateTimeField(auto_now_add=True)
def __str__(self):
return f"{self.student} enrolled in {self.course}"

Step-04: Run the below command to create the model:-
python manage.py makemigrations
python manage.py migrate
Step-05: Inside admin.py file define model:-
from django.contrib import admin
from .models import Student, Course
class StudentAdmin(admin.ModelAdmin):
list_display = ('first_name', 'last_name', 'email', 'phone_number')
search_fields = ('name', 'email', 'phone_number')
list_filter = ('courses',)
class CourseAdmin(admin.ModelAdmin):
list_display = ('name',)
search_fields = ('name',)
admin.site.register(Student, StudentAdmin)
admin.site.register(Course, CourseAdmin)
Step-06: Inside urls.py file define model:-
⦿ Open the file in your Django project directory (project/urls.py).
⦿ Import the view function at the top of the file.
⦿ Add new URL pattern to the urlpatterns list.
from django.urls import path
from django.contrib import admin
from django.shortcuts import redirect
urlpatterns = [
path('admin/', admin.site.urls),
path('', lambda request: redirect('admin/', permanent=True)),
]

Step-07: Create a superuser:-
⦿ Fill all the details after running below command…
python manage.py createsuperuser

Step-08: Run Your Project:-
⦿ Now setup is completed you can run your project using below command.
⦿ The terminal will display the URL where the development server is running, typically type http://127.0.0.1:8000/admin
python manage.py runserver



