12. Develop a registration page for student enrolment as done in Module 2 but without page refresh using AJAX.
Step-01: Create new folder:-
⦿ In the same project folder whatever we made earlier create again one new app name called as ‘registration’ using below command.
cd project
python manage.py startapp registration
Step-02: Add the ‘registration’ to the installed_apps list:-
⦿ locate the settings.py file (usually located in the project directory) and open it.
⦿After then add your app name in install_apps list as per below image.
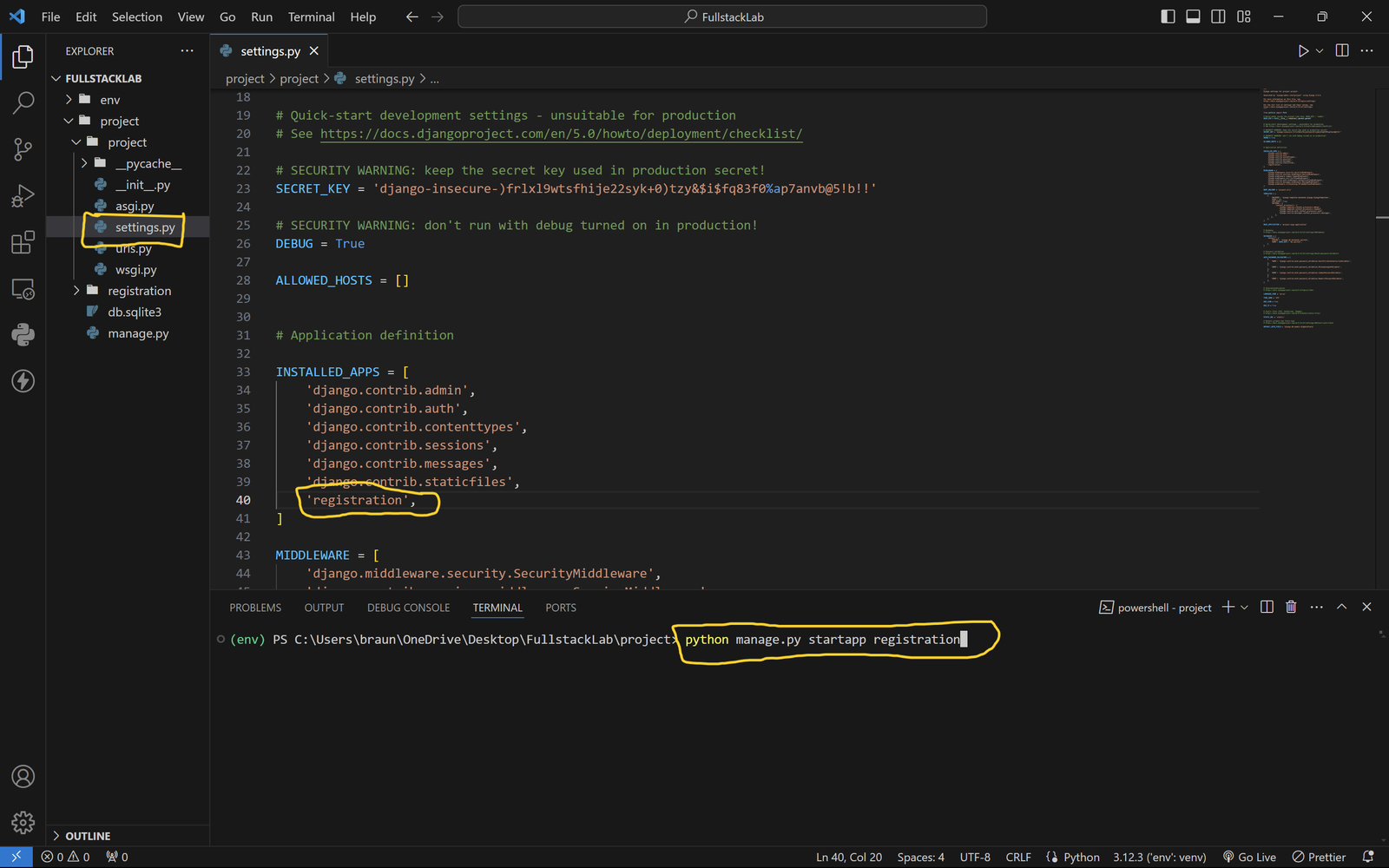
Step-03: Inside models.py file create a model:-
from django.db import models
class Student(models.Model):
fname = models.CharField(max_length=255, null=True)
lname = models.CharField(max_length=255, null=True)
email = models.EmailField(null=True, unique=True)
topic = models.CharField(max_length=255, null=True,)
languages_used = models.CharField(max_length=255, null=True)
duration = models.IntegerField(null=True)
def __str__(self):
return self.topic

Step-04: Run the below command to create the model:-
python manage.py makemigrations
python manage.py migrate
Step-05: Create forms.py file inside the ‘registration’ folder:-
⦿ Open the forms.py file in your Django project directory (registration/forms.py).
⦿ Create a new form that will handle the request using below command.
from django import forms
from .models import Student
class StudentForm(forms.ModelForm):
class Meta:
model = Student
fields = ('fname', 'lname', 'email', 'topic', 'languages_used', 'duration')
labels = {
'topic': 'Project Name',
'languages_used': 'Languages Used',
'duration': 'Duration (in days)',
'fname': 'First Name',
'lname': 'Last Name',
'email': 'Email'
}

Step-06: Inside views.py file create a function:-
⦿ Open the views.py file in your Django project directory (registration/views.py).
⦿ Import the required modules at the top of the file.
⦿ Create a new view function that will handle the request and display the data in the templates.
from django.shortcuts import render
from django.http import JsonResponse
from .models import Student
from .forms import StudentForm
def student_register(request):
if request.method == 'POST':
form = StudentForm(request.POST)
if form.is_valid():
form.save()
return JsonResponse({'message': 'Student registered successfully!'})
else:
return JsonResponse({'message': ' This email is already registered' })
else:
form = StudentForm()
return render(request, 'student_register.html', {'form': form})
def register_list(request):
students = Student.objects.all()
return render(request, 'register_list.html', {'students': students})

Step-07: Create a template:-
⦿ Right click on ‘registration’ folder, create a new folder named templates.
⦿ Inside the templates folder, create a new file named base.html.
⦿ Inside the templates folder, create a new file named student_register.html.
⦿ Inside the templates folder, create a new file named register_list.html.
⦿ Copy all the different different html file code and paste into all different html file to display the user interface.
base.html
<!DOCTYPE html>
<html>
<head>
<title>Student Registration</title>
<style>
* {
padding: 0;
margin: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
}
header {
justify-content: center;
align-items: center;
display: flex;
background: #000;
color: #fff;
padding: 15px;
text-align: center;
}
header nav ul {
list-style: none;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
}
header nav li {
margin-right: 20px;
}
header nav a {
transition: all 0.3s ease;
font-weight: 600;
color: #fffd3c;
text-decoration: none;
}
header nav li a:hover {
color: lightgreen;
}
footer p {
font-weight: 600;
}
main {
margin: auto;
display: flex;
flex-direction: column;
align-items: center;
padding: 20.5px;
}
#message {
color: blue;
text-align: center;
padding-top: 18px;
}
form {
box-shadow: rgba(0, 0, 0, 0.1) 0px 0px 5px 0px,
rgba(0, 0, 0, 0.1) 0px 0px 1px 0px;
width: 30%;
margin: 0 auto;
padding: 20px 20px 10px 20px;
background: #fff;
border-radius: 10px;
}
form h1, h2 {
font-size:20px;
text-align: center;
margin-bottom: 12px;
border-radius: 10px;
padding: 10px;
color: #fff;
background: #000;
font-size: 24px;
}
label {
display: block;
margin-bottom: 10px;
}
input,
textarea {
border-radius: 10px;
width: 100%;
padding: 10px;
margin-bottom: 15px;
border: 1px solid #ccc;
}
button[type="submit"] {
font-size: 16px;
font-weight: 600;
width: 100%;
background-color: #0b742e;
color: #fff;
padding: 14px;
border: none;
border-radius: 10px;
cursor: pointer;
}
button[type="submit"]:hover {
background-color: #3e8e41;
}
footer {
background: #000;
color: #fff;
padding: 15px;
text-align: center;
}
.project-list {
box-shadow: rgba(60, 64, 67, 0.3) 0px 1px 2px 0px,
rgba(60, 64, 67, 0.15) 0px 1px 3px 1px;
border-radius: 10px;
background: #fff;
list-style: none;
padding: 20px;
margin: 0;
}
span {
font-weight: bold;
}
ul.project-list h1 {
font-size: 25px;
border-radius: 10px;
padding: 10px;
margin-bottom: 20px;
background: #000;
color: #fff;
text-align: center;
}
.project-list li {
border: 1px solid #00000054;
transition: all 0.4s ease;
border-radius: 10px;
margin-bottom: 8.3px;
background: #fff;
box-shadow: rgba(14, 63, 126, 0.06) 0px 0px 0px 1px,
rgba(42, 51, 70, 0.03) 0px 1px 1px -0.5px,
rgba(42, 51, 70, 0.04) 0px 2px 2px -1px,
rgba(42, 51, 70, 0.04) 0px 3px 3px -1.5px,
rgba(42, 51, 70, 0.03) 0px 5px 5px -2.5px,
rgba(42, 51, 70, 0.03) 0px 10px 10px -5px,
rgba(42, 51, 70, 0.03) 0px 24px 24px -8px;
padding: 15px;
}
.project-list li:hover {
background: #ffecf2a6;
box-shadow: rgba(14, 63, 126, 0.06) 0px 0px 0px 1px,
rgba(42, 51, 70, 0.03) 0px 1px 1px -0.5px,
rgba(42, 51, 70, 0.04) 0px 2px 2px -1px,
rgba(42, 51, 70, 0.04) 0px 3px 3px -1.5px,
rgba(42, 51, 70, 0.03) 0px 5px 5px -2.5px,
rgba(42, 51, 70, 0.03) 0px 10px 10px -5px,
rgba(42, 51, 70, 0.03) 0px 24px 24px -8px;
}
.project-info {
display: flex;
flex-direction: column;
align-items: center;
}
.project-info h2 {
font-weight: bold;
margin-top: 0;
}
.project-info p {
margin-bottom: 10px;
}
.project-info span {
font-weight: bold;
margin-right: 10px;
}
.project-list li:hover:before {
color: #23527c;
}
</style>
</head>
<body>
<header>
<nav>
<ul>
<li><a href="{% url 'student_register' %}">Student Register</a></li>
<li><a href="{% url 'register_list' %}">Register List</a></li>
</ul>
</nav>
</header>
<main>{% block content %}{% endblock %}</main>
<footer>
<p>
Designed by Braham Kumar | Copyright © 2024 vtucode | All Right Reserved...
</p>
</footer>
</body>
</html>
student_register.html
{% extends 'base.html' %}
{% block content %}
<form id="studentForm" method="post">
<h2>Student Registration</h2>
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Register</button>
<div id="message"></div>
</form>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$('#studentForm').submit(function(event) {
event.preventDefault();
$.ajax({
type: 'POST',
url: '{% url "student_register" %}',
data: $(this).serialize(),
success: function(response) {
$('#message').html('<p>' + response.message + '</p>');
$('#studentForm')[0].reset();
},
error: function(response) {
$('#message').html('<p>Error: ' + response.responseJSON.error + '</p>');
}
});
});
});
</script>
{% endblock %}
register_list.html
{% extends 'base.html' %}
{% block content %}
<ul class="project-list">
<h1>Registered students</h1>
{% for student in students %}
<li>
<span>Name:</span> {{ student.fname }} {{ student.lname }}<br>
<span>Email:</span> {{ student.email }}<br>
<span>Project Name:</span> {{ student.topic }}<br>
<span>Languages Used:</span> {{ student.languages_used }}<br>
<span>Duration:</span> {{ student.duration }} days
</li>
{% empty %}
<li>No students registered yet.</li>
{% endfor %}
</ul>
{% endblock %}
Step-08: Add URLs path in the project’s URL patterns:-
⦿ Open the file in your Django project directory (project/urls.py).
⦿ Import the view function at the top of the file.
⦿ Add new URL pattern to the urlpatterns list.
from django.urls import path
from registration import views
urlpatterns = [
path('', views.student_register, name='student_register'),
path('register_list', views.register_list, name='register_list'),
]

Step-09: Run Your Project:-
⦿ Now setup is completed you can run your project using below command.
⦿ The terminal will display the URL where the development server is running, typically http://127.0.0.1:8000/.
⦿ Copy the URL from the terminal and paste it into your web browser’s address bar to see the output web page.
python manage.py runserver


